Installation instructions
Eclipse interface
Command line interface
Tutorial
Eclipse interface
Make sure that you have JRE 1.8 configured in Eclipse.
Quick start
You can use an example wizard to add an example project with demo models into your workspace.
- Select File | New | Example... | xUML-RT Examples > xUML-RT State Machine Examples
- Click Next.
- Click Finish.
- Open any of the models in the newly created xUML-RTExamples project
- Open the state machine diagram in Papyrus.
- Debug the corresponding pre-created launch configuration and see the animation on the state machine and the console log.
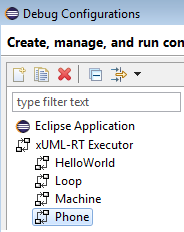
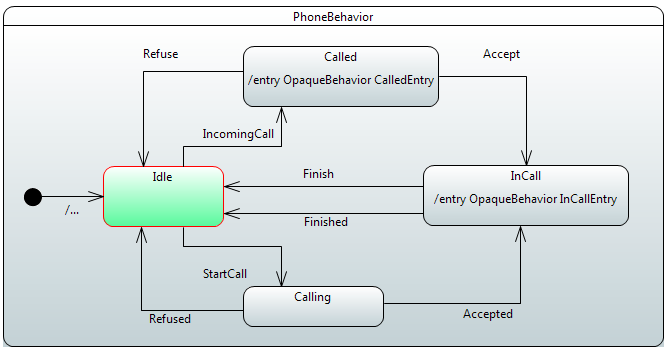
- You can add breakpoints to the state machines by right clicking a state or transition on the diagram (or in the model explorer), then selecting Moka > Debug > Toggle breakpoint.
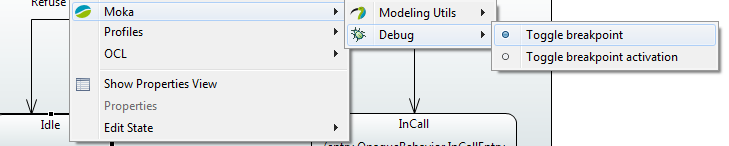
- Standard debug controls of the Debug view (pause, step, resume, stop) are supported.
Create a new project of type xUML-RT Project
- In Project Explorer select New | Project... | Papyrus > xUML-RT Project
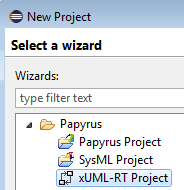
- Set project name
- Press the Finish button.
Adding a new EMF-UML2 model
You can add a new model to the xUML-RT Project:
- Select
File | New | Other...
menu item.
- Select
Papyrus > Papyrus Model
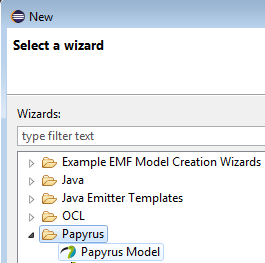
- See the Papyrus documentation on how to edit models.
- All action code in the model (operation body, entry/exit actions, transition effects) must be written in opaque beaviors (as classifier behaviors) in the rAlf action language.
- See the Features section for the list of model elements currently supported by the Model Executor.
Alternatively, you can also copy an existing model into the project.
Pre-requisite for execution: implement a static feed function
In order to execute a model you need to implement a static feed function which contains action
code that makes your model move (e.g. create object instances, sends signals).
Phone p = new Phone();
send new Refused() to p;
send new IncomingCall() to p;
Pre-requisite for execution: resolve all static validation errors
When the structure of a model fails a static validation rule, the validator assigns an error marker to the problematic model element.
As the execution of models is based on code generation, the system only works with valid models. Therefore only valid models can be
executed either in run or debug mode. However, there are some validation errors that may not affect the code generation procedure.
To enable code generation without respect to any validation errors, change the value of the Code should not be generated:
field to always generate
on the xUML-RT Executor
page between the project properties.
The default value is on errors
.
Execute an xUML-RT Project
In order to run a model, you need to create a run configuration.
- Select
Run | Debug Configurations... | xUML-RT Executor | New
- Set the following:
- Name of the configuration
- Set Select model: the
.uml
file of the model
- Set Select class: class containing an operation that shall be called to run the model
- Set Select feed function: the operation of the selected class to be called
- In order to enable/disable logging select tab
Tracing and Logging
and select/deselect the Enable logging
check box.
- Press Run
Replaying a previous model execution
In order to replay a previous model execution you need to enable tracing in the run configuration. To enable/disable tracing open the corresponding run configuration, select tab Tracing and Logging
and select/deselect the Enable tracing
check box. Trace files are stored in folder traces
under the project folder.
If tracing is enabled, after a model execution is terminated, a trace file is generated in folder traces
. You can replay a previous execution by opening its run configuration, and under the Tracing and Logging
tab selecting the Enable replay
check box and setting the trace file. Replay starts after you press the Run
or Debug
button.
Adding breakpoints
You can add breakpoints to the state machines by right clicking a state or transition on the diagram (or in the model explorer), then selecting Moka | Debug | Toggle breakpoint
.
Note that breakpoints can be added before or even during model execution.
Debugging
In order to debug the model you need to create a run configuration and run it in debug mode. Standard debug controls of the Debug
view (pause, step, resume, stop) are supported.
Animation configuration:
- Auto-display diagram: Open that diagram on which a state transition is happening. In order to enable/disable auto-display diagram switch to
Debug
perspective and select/deselect Opens diagram automatically
check box in Animation Configuration
view.
- Animation: During model execution the model executor can highlight those states and transitions that are being traversed; it is called animation. In order to enable/disable a animation switch to
Debug
perspective and check/uncheck Animate
in Animation Configuration
view.
- Animation delay: You can also fine-tune the speed of animation. Switch to
Debug
perspective and adjust the animation delay in Animation Configuration
view.
Command line interface
- Ask for all supported options:
java -jar xumlrt-executor-cli-0.8.0.jar --help
- Setup a model for execution:
java -jar xumlrt-executor-cli-0.8.0.jar --root gen --setup model.uml
Note: Use java from a JDK in order to ensure successful compilation of the generated Java files.
- Execute the model:
java -jar xumlrt-executor-cli-0.8.0.jar --root gen --execute ClassName opName --logger minimal
External communication
Beginning with release 0.4.0 Model executor supports calling external Java classes, i.e. a Java classes which are not part of the executable model. The implementation is based on the introduction of external entities.
From the model's point of view, an external entity is an abstract class marked with the «externalEntity»
stereotype. These classes must have only static methods, which will be implemented by external Java classes. When an external entity method is called from the action code in the model, the call will be dispatched to an instance of the selected Java class. The fully qualified name of the implementation class must be provided as an attribute of the stereotype. By default a new instance will be created by the executor every time it dispatches an external entity method call. It is also possible to configure an external entity to create a lazy singleton, which will be instantiated at the first method call. Then every other invocations will use the same Java class instance of that external entity. This behavior can also be specified a stereotype attribute. Using the «externalEntity»
stereotype on a class will add several constraints to its validation process, which are described in a separate section below. These constraints are ensuring that a valid Java interface could be generated from the external entity definition. The implementation class of an external entity then must implement its generated interface.
It is also possible to send events to the state machine of an active class from an external entity, which enables two-way communication with native code. Active classes which are able to receive events from external entities through receptions must be marked with the «callable»
stereotype. Then for each callable class a Java proxy class will be generated. An external entity method then could have parameters that have «callable»
types. The external entity implementation could use these references to call the appropriate receptions on active class instances. Validation of callable classes is also constrained to ensure the class name and the reception names are valid Java identifiers. For more information on constraints, see their separate section below. Primitive types are also allowed as parameters, and also as return values. The multiplicity of each parameter and return value must be exactly 1.
Steps to create an external entity with a callable active class
- Apply the xUML-RT registered profile on the model root. This could be done from its properties view, under the Profile tab.
- Create a class which has a state machine as its owned behavior.
- If the external entity will send signals to the state machine, apply the
«callable»
stereotype on the class. Stereotype applications could also be done under the Profile tab on the properties view of the selected element.
- Add the required receptions to the class according to the naming constraints below. These will be accessible from external entities to send events for a state machine instance.
- Create an abstract class for an external entity according to the constraints below, and apply the
«externalEntity»
stereotype on it.
- Specify the fully qualified Java implementation class name for the external entity with the
class
stereotype attribute.
- Select an instantiation policy using the
type
stereotype attribute. Stateless
will dispatch every external entity method invocation to a new instance of the implementation class, while Singleton
will use a single instance which will be created by the executor at the first method call.
- Add static methods to the external entity. These methods must not have any parameters if they don't send any event to any state machine.
- To implement two-way communication, create one or more methods in the external entity class which have a parameter with input direction. The parameter must conform to the constraints detailed in the next section — so it must refer to a
«callable»
class instance, or a primitive type.
- Implement the specified external entity class in Java. The simplest way is to extend the source path of the current xUML-RT project in its properties window with another source directory. It could be done under the Java Build Path / Source tab.
- The implementation class must be placed into this source directory, and have the same fully qualified name as it was specified earlier in the
«externalEntity»
stereotype's class
attribute.
- When an xUML-RT project is being built, it generates a Java interface for each external entity with exactly the same name as its class name. Every implementation class must explicitly implement that generated Java interface. When a method refers to a callable class instance as its parameter, the receptions available could be invoked from Java through that reference.
- Call the external entity in your action code. The external entity operations could be referenced with the name of the entity and with the method name, separated by double colons (::). For example, when the external entity name is
Entity
, and the method name is method
, the following statement could be used from action code: Entity::method();
- When the given external entity method supports two-way communication, the current
«callable»
class instance could be provided as a parameter to the call from the action code as Entity::method(instanceWithStateMachine);
Stereotype constraints
The model must be explicitly validated to trigger these constraints. This could be requested for instance from the context menu on the model root in the Model Explorer view of Papyrus. The next concepts are used in the constraints below:
- Java identifier
- A string matching the regular expression
[\p{L}_$][\p{L}\p{N}_$]*
. It must start with a unicode letter or an underscore character, or either with a dollar sign. The follwoing characters could also contain numbers. A valid identifier must be at least one character long according to this constraint.
- Fully qualified Java class name
- Consists of a single or multiple Java identifiers, separated by dots.
Constraints on classes marked with «externalEntity»
- The class name must be a Java identifier.
- The class must be abstract.
- It must not have any attribute.
- The implementation class name referenced in the
class
stereotype attribute must be a Fully qualified Java class name.
- Only static operations are allowed.
- All operation names must be valid Java identifiers.
- An operation could have any number of input parameters, and at most one return parameter.
If parameters are present in the signature of an external entity operation, they must conform to the following constraints:
- It is an input or a return parameter.
- The parameter name is a Java identifier.
- Multiplicity of the parameter is one ([1..1]).
- The type of the parameter is a class which is marked with the
«callable»
stereotype or a primitive type.
Constraints on classes marked with «callable»
- The class name must be a Java identifier.
- All reception names must be Java identifiers.
Features
Classes
- Any number of classes can be created
- A class can have a state machine
- A class with a state machine can receive events
- Class properties are supported
- Class-level and instance-level attributes
- Multiplicity is supported
- Attributes with multiple values can be ordered or unordered unique/nonunique
- Class operations are supported
- Class-level and instance-level operations
- Operation behavior can be specified by opaque behavior
- Return type of operations is supported
- Return type can have multiplicity
- Return with multiple values can be ordered or unordered unique/nonunique
- Parameters of operations are supported
- Parameters have direction of in/out/inout
- Multiplicity is supported
- Arguments with multiple values can be ordered or unordered unique/nonunique
- Class associations are supported
- Association ends must be owned by the association (not the default behavior of Papyrus)
- Associations must be binary
- Association classes are supported, association classes can have attributes, operations, associations, etc. like any other class.
- Navigation over associations
- Class receptions are supported
- Receptions have parameters that will be passed to the created event
- Therefore receptions can only have primitive types as their parameters
Inheritance
- Classes can inherit from other classes
- Support for multiple inheritance
- No self-inheritance, multiple inheritance from the same class, or loops in the inheritance graph
- Attributes, operations, receptions, associations and state machines are inherited
- If more than one ancestors of a class define state machines, it should also define a state machine (cannot choose which to inherit)
- Operations can override other operations
- Only instance-level operations can be overridden
- Indirect overriding is supported (g overrides f, h overrides g)
- For a given inherited method in a given class there must be only one (indirect) overrider that is not overridden
Events
- Signal events are supported
- Event types are erased for model execution
Signals
- Signal attributes are supported
- Signal attributes must have primitive types
- Signal attributes can have multiplicity
- Signal attributes with multiple values can be ordered or unordered unique/nonunique
State machines
- The state machine must have exactly one region
- SM's have states and transitions
- States can be normal states, initial states or termination states
- Entry and exit actions are supported on states
- Transitions can have an effect
- The state machine must have an initial state and a transition from the initial state to a normal state that has no event on it
- When the state machine starts, the effect of the initial transition and the entry action of the first normal state is executed
- When the control reaches the termination state the class with the state machine will not receive any more events
Action code
- Sending and receiving signals.
- Accessing operation attributes.
- Creating new class instances.
- Basic arithmetic: +, -, /, *
Communication with external entities
Calling external Java classes, i.e. a Java classes which are not part of the executable model.